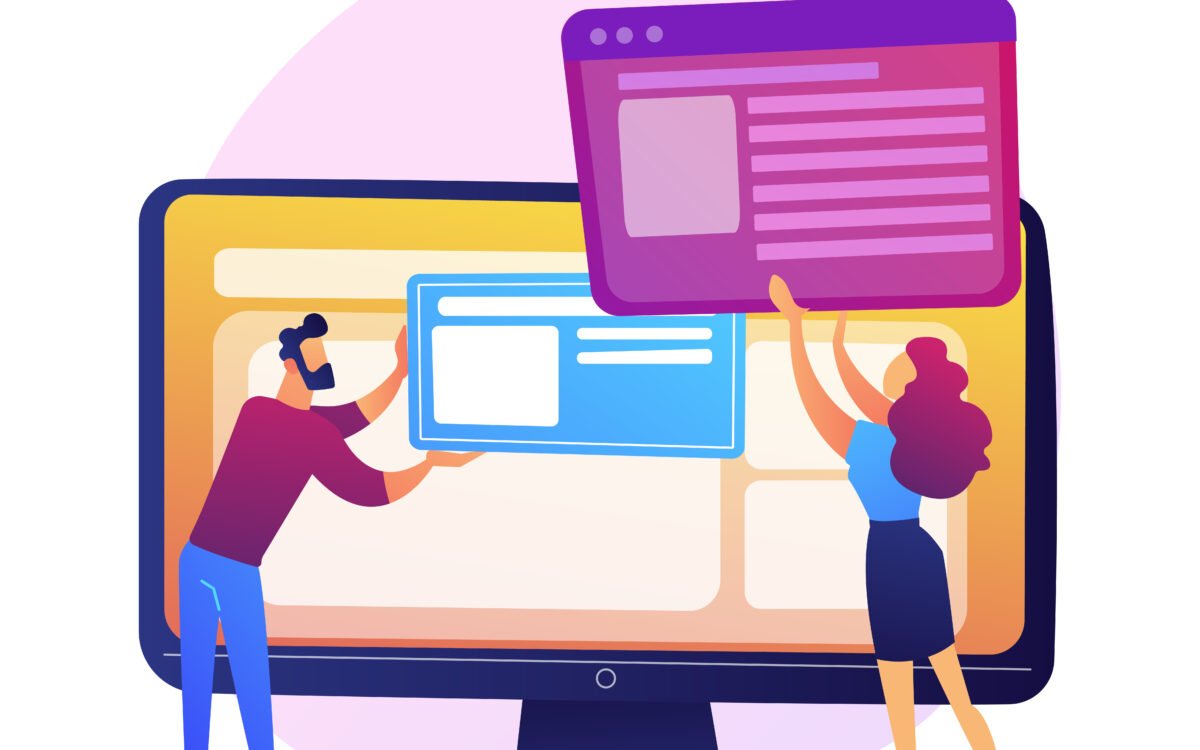
Advanced Headless Browser Testing with Selenium
Selenium is a robust web automation testing tool that allows you to automate interactions with web applications across different browsers. One of its most significant advantages is the ability to run tests in headless mode, where the browser operates without a graphical user interface (UI). This tutorial will walk you through the concept of headless browser testing, how it improves performance, and how to configure it for Chrome, Firefox, and Edge using your website qatechxperts as an example.
What is Headless Browser Testing?
Headless Browser Testing refers to running automated tests on a browser without the user interface. This means that the web pages are loaded and interacted with, but no graphical interface is displayed. This allows for faster test execution, as the browser does not need to render the UI components such as HTML, CSS, and JavaScript.
Headless browsers are useful in scenarios where you need to perform automation on servers, where there is no GUI, or in environments where a faster execution time is critical, such as in Continuous Integration/Continuous Deployment (CI/CD) pipelines. Selenium makes it easy to run tests in headless mode for Chrome, Firefox, and Edge browsers.
Why is Headless Execution Important?
Headless execution is particularly important because it enhances the speed and efficiency of automation testing:
- Speed: Tests run faster because rendering visual components is skipped. This speeds up the entire automation process.
- Resource Efficiency: Without the need to render a graphical interface, headless browsers use less memory and CPU resources, making them ideal for large-scale automated testing environments.
- CI/CD Integration: Headless testing can be seamlessly integrated into CI/CD pipelines where execution time and resource usage are a concern. It ensures that tests are executed quickly and consistently in environments without GUIs.
- Parallel Testing: Since headless browsers consume fewer resources, they are perfect for running tests concurrently. This allows for parallel testing, improving test throughput.
Running Selenium Tests in Headless Mode for Chrome
Chrome supports headless mode from version 59 onwards. Selenium can configure Chrome to run in headless mode using the ChromeOptions
class. Here is a step-by-step guide to running headless tests with Chrome using your website qatechxperts:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.testng.Assert;
import org.testng.annotations.Test;
public class ChromeHeadlessTest {
WebDriver driver;
@Test
public void verifyTitle() {
// Set up ChromeOptions for headless mode
ChromeOptions options = new ChromeOptions();
options.addArguments("--headless"); // Enabling headless mode
// Initialize ChromeDriver with options
driver = new ChromeDriver(options);
// Navigate to the website
driver.get("https://www.qatechxperts.com");
// Output the title
System.out.println("Page title: " + driver.getTitle());
// Assert the title
Assert.assertEquals(driver.getTitle(), "QA Tech Xperts | Leading Quality Assurance Services");
// Quit the driver
driver.quit();
}
}
Test Output
The output of this test should display the page title on the console, confirming that Chrome has successfully executed the test in headless mode:
Test Case | Expected Result | Actual Result |
---|---|---|
Verify Page Title | “QA Tech Xperts | Leading Quality Assurance Services” | “QA Tech Xperts | Leading Quality Assurance Services” |
Running Selenium Tests in Headless Mode for Firefox
Firefox introduced headless mode in version 56. To run Firefox in headless mode using Selenium, the FirefoxOptions
class is used. Below is the example code for headless testing on Firefox using your website qatechxperts:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.firefox.FirefoxOptions;
import org.testng.Assert;
import org.testng.annotations.Test;
public class FirefoxHeadlessTest {
WebDriver driver;
@Test
public void verifyTitle() {
// Set up FirefoxOptions for headless mode
FirefoxOptions options = new FirefoxOptions();
options.addArguments("-headless");
// Initialize FirefoxDriver with options
driver = new FirefoxDriver(options);
// Navigate to the website
driver.get("https://www.qatechxperts.com");
// Output the title
System.out.println("Page title: " + driver.getTitle());
// Assert the title
Assert.assertEquals(driver.getTitle(), "QA Tech Xperts | Leading Quality Assurance Services");
// Quit the driver
driver.quit();
}
}
Test Output
The output for this Firefox headless test will be similar to Chrome, displaying the page title in the console:
Test Case | Expected Result | Actual Result |
---|---|---|
Verify Page Title | “QA Tech Xperts | Leading Quality Assurance Services” | “QA Tech Xperts | Leading Quality Assurance Services” |
Running Selenium Tests in Headless Mode for Edge
Edge also supports headless mode starting from version 79. Similar to Chrome and Firefox, Selenium allows configuring headless mode for Edge with the EdgeOptions
class:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.edge.EdgeDriver;
import org.openqa.selenium.edge.EdgeOptions;
import org.testng.Assert;
import org.testng.annotations.Test;
public class EdgeHeadlessTest {
WebDriver driver;
@Test
public void verifyTitle() {
// Set up EdgeOptions for headless mode
EdgeOptions options = new EdgeOptions();
options.addArguments("--headless");
// Initialize EdgeDriver with options
driver = new EdgeDriver(options);
// Navigate to the website
driver.get("https://www.qatechxperts.com");
// Output the title
System.out.println("Page title: " + driver.getTitle());
// Assert the title
Assert.assertEquals(driver.getTitle(), "QA Tech Xperts | Leading Quality Assurance Services");
// Quit the driver
driver.quit();
}
}
Test Output
For Edge headless testing, the output is similar to the others, where the page title is displayed in the console:
Test Case | Expected Result | Actual Result |
---|---|---|
Verify Page Title | “QA Tech Xperts | Leading Quality Assurance Services” | “QA Tech Xperts | Leading Quality Assurance Services” |
Comparison: Headless Browser vs Regular Browser
When choosing between headless and regular browser testing, there are several factors to consider. Below is a comparison:
Factor | Headless Browser | Regular Browser |
---|---|---|
Performance | Faster execution, fewer resources required | Slower due to rendering of the UI |
Visual Debugging | No visual output available | Allows visual debugging directly on the screen |
Resource Consumption | Consumes less memory and CPU | Requires more resources due to rendering |
Ideal Use Case | CI/CD pipelines, automated backend testing, performance testing | UI testing, manual testing, debugging |
Test Speed | 2x faster than regular browsers | Slower |
Conclusion
Headless browser testing is a powerful tool that speeds up test execution and reduces resource consumption. It’s ideal for performance testing, web scraping, and CI/CD integrations, but not suitable when visual verification is required. Regular browsers, on the other hand, are necessary for UI testing and visual debugging.